coreData数据最终的存储类型可以是:SQLite数据库、XML、二进制、内存里、自定义的数据类型。
和SQLite区别:只能取出整个实体记录,然后分解,之后才能得到实体的某个属性。
1、创建工程勾选use coreData选项。
AppDelete.swift中自动生成一些方法:
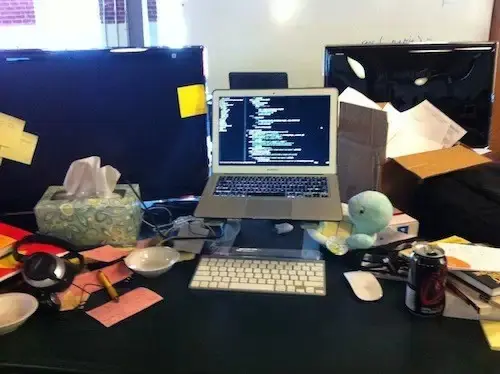
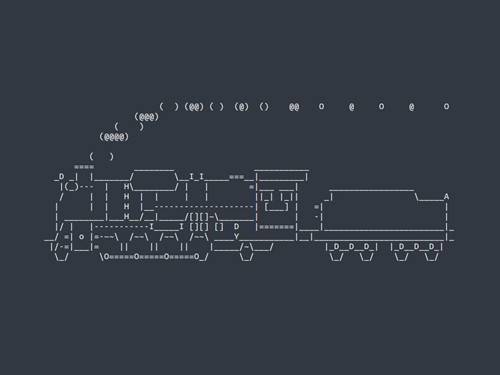
1 // MARK: - Core Data stack
2
3 lazy var applicationDocumentsDirectory: NSURL = {
4 // The directory the application uses to store the Core Data store file. This code uses a directory named "com.LMY.LMYCoreData" in the application's documents Application Support directory.
5 let urls = NSFileManager.defaultManager().URLsForDirectory(.DocumentDirectory, inDomains: .UserDomainMask)
6 return urls[urls.count-1]
7 }()
8
9 lazy var managedObjectModel: NSManagedObjectModel = {
10 // The managed object model for the application. This property is not optional. It is a fatal error for the application not to be able to find and load its model.
11 let modelURL = NSBundle.mainBundle().URLForResource("LMYCoreData", withExtension: "momd")!
12 return NSManagedObjectModel(contentsOfURL: modelURL)!
13 }()
14
15 lazy var persistentStoreCoordinator: NSPersistentStoreCoordinator = {
16 // The persistent store coordinator for the application. This implementation creates and returns a coordinator, having added the store for the application to it. This property is optional since there are legitimate error conditions that could cause the creation of the store to fail.
17 // Create the coordinator and store
18 let coordinator = NSPersistentStoreCoordinator(managedObjectModel: self.managedObjectModel)
19 let url = self.applicationDocumentsDirectory.URLByAppendingPathComponent("SingleViewCoreData.sqlite")
20 var failureReason = "There was an error creating or loading the application's saved data."
21 do {
22 try coordinator.addPersistentStoreWithType(NSSQLiteStoreType, configuration: nil, URL: url, options: nil)
23 } catch {
24 // Report any error we got.
25 var dict = [String: AnyObject]()
26 dict[NSLocalizedDescriptionKey] = "Failed to initialize the application's saved data"
27 dict[NSLocalizedFailureReasonErrorKey] = failureReason
28
29 dict[NSUnderlyingErrorKey] = error as NSError
30 let wrappedError = NSError(domain: "YOUR_ERROR_DOMAIN", code: 9999, userInfo: dict)
31 // Replace this with code to handle the error appropriately.
32 // abort() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
33 NSLog("Unresolved error \(wrappedError), \(wrappedError.userInfo)")
34 abort()
35 }
36
37 return coordinator
38 }()
39
40 lazy var managedObjectContext: NSManagedObjectContext = {
41 // Returns the managed object context for the application (which is already bound to the persistent store coordinator for the application.) This property is optional since there are legitimate error conditions that could cause the creation of the context to fail.
42 let coordinator = self.persistentStoreCoordinator
43 var managedObjectContext = NSManagedObjectContext(concurrencyType: .MainQueueConcurrencyType)
44 managedObjectContext.persistentStoreCoordinator = coordinator
45 return managedObjectContext
46 }()
47
48 // MARK: - Core Data Saving support
49
50 func saveContext () {
51 if managedObjectContext.hasChanges {
52 do {
53 try managedObjectContext.save()
54 } catch {
55 // Replace this implementation with code to handle the error appropriately.
56 // abort() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
57 let nserror = error as NSError
58 NSLog("Unresolved error \(nserror), \(nserror.userInfo)")
59 abort()
60 }
61 }
62 }
63
64 }
lazy var applicationDocumentsDirectory: NSURL = {// The directory the application uses to store the Core Data store file. This code uses a directory named "com.LMY.LMYCoreData" in the application's documents Application Support directory.let urls = NSFileManager.defaultManager().URLsForDirectory(.DocumentDirectory, inDomains: .UserDomainMask)return urls[urls.count-1]}()
表示应用程序沙盒下的Documents目录路径。
选中coreData.xcdatamodeld文件,添加实体并命名,选中实体添加相关的属性。
可以切换Editor Style按钮查看实体的关系图。
2、为每个实体生成一个N SManagedObject子类,通过类的成员属性来访问和获取数据。选择CoreData项下面N SManagedObject subClass类型文件,生成该实体同名的类User.swift。
在User.swift对象类中添加一句代码:
//objc特性告诉编译器该声明可以在Objective-C代码中使用
@objc(User)
User对象创建以后,通过对象来使用coreData。
3、插入数据需要以下步骤:
1)通过appdelegate单例来获取管理的数据的上下文对象,操作实际内容。
//获取管理的数据上下文对象let app = UIApplication.sharedApplication().delegate as! AppDelegatelet context = app.managedObjectContext
2)通过NSEntityDescription.insertNewObjectForEntityForName方法来创建实体对象。
3)给实体对象赋值。
4)context.save保存实体对象。
具体代码如下:
//获取管理的数据上下文对象let app = UIApplication.sharedApplication().delegate as! AppDelegatelet context = app.managedObjectContext//创建User对象let oneUser = NSEntityDescription.insertNewObjectForEntityForName("User", inManagedObjectContext: context) as! User//对象赋值oneUser.userID = 2oneUser.userEmail = "18500@126.com"oneUser.userPawd = "18500"//保存do{try context.save()}catch let error as NSError {print("不能保存:\(error)")//如果创建失败,error 会返回错误信息}
4、查询操作具体分为以下几步:
1)NSFetchRequest方法来声明数据的请求,相当于查询语句。
2)NSEntityDescription.entityForName方法声明一个实体结构,相当于表格结构。
3)NSPredicate创建一个查询条件,并设置请求的查询条件。
4)context.executeFetchRequest执行查询操作。
5)使用查询出来的数据。
具体代码如下:
//------- 查询//声明数据的请求let fetchRequest:NSFetchRequest = NSFetchRequest()fetchRequest.fetchLimit = 10// 限定查询结果的数量fetchRequest.fetchOffset = 0// 查询的偏移量//声明一个实体结构let entity:NSEntityDescription? = NSEntityDescription.entityForName("User", inManagedObjectContext: context)//设置数据请求的实体结构fetchRequest.entity = entity//设置查询条件let predicate = NSPredicate(format: "userID = '2'", "")fetchRequest.predicate = predicate//查询操作do{let fetchedObjects:[AnyObject]? = try context.executeFetchRequest(fetchRequest)//遍历查询的结果for info:User in fetchedObjects as! [User] {print("userID = \(info.userID)")print("userEmail = \(info.userEmail)")print("userPawd = \(info.userPawd)")print("++++++++++++++++++++++++++++++++++++++")}}catch let error as NSError {print("查询错误:\(error)")//如果失败,error 会返回错误信息}
5、修改数据库方法,使用很简单,将查询出来的对象进行重新赋值,然后再使用context.save方法重新保存即可实现。
具体代码如下:
//----------修改do{let fetchedObjects:[AnyObject]? = try context.executeFetchRequest(fetchRequest)//遍历查询出来的所有对象for info:User in fetchedObjects as! [User] {print("userID = \(info.userID)")print("userEmail = \(info.userEmail)")print("userPawd = \(info.userPawd)")print("++++++++++++++++++++++++++++++++++++++")//修改邮箱info.userEmail = "18500_junfei521@126.com"//重新保存do{try context.save()}catch let error as NSError {print("不能保存:\(error)")//如果失败,error 会返回错误信息
}}}catch let error as NSError {print("修改失败:\(error)")//如果失败,error 会返回错误信息}
6、删除操作使用context.deleteObject方法,删除某个对象,然后使用context.save方法保存更新到数据库。
具体代码如下:
//---------删除对象do{let fetchedObjects:[AnyObject]? = try context.executeFetchRequest(fetchRequest)for info:User in fetchedObjects as! [User]{context.deleteObject(info)}//重新保存-更新到数据库do{try context.save()}catch let error as NSError {print("删除后保存失败:\(error)")//如果失败,error 会返回错误信息
}}catch let error as NSError {print("删除后保存失败:\(error)")//如果失败,error 会返回错误信息}
7、可以获取项目安装的路径
//获取路径let homeDirectory = NSHomeDirectory()print(homeDirectory)