作者:鄙人灵魔世界 | 来源:互联网 | 2023-09-10 20:56
MyTarget:Getonephotofromcameraorgallery,thensetittoimageViewA从相机或图库中获取一张照片,然后将其设置
My Target:
- Get one photo from camera or gallery, then set it to imageViewA
从相机或图库中获取一张照片,然后将其设置为imageViewA
- Add a draggable mask imageViewB on top of imageViewA
在imageViewA上添加一个可拖动的蒙版imageViewB
- Users drag the mask imageViewB to anywhere in imageViewA
用户将遮罩imageViewB拖动到imageViewA中的任何位置
- Users then click the save button
然后用户单击“保存”按钮
- A combined bitmap of imageViewA and imageViewB should be created
应创建imageViewA和imageViewB的组合位图
My Problem:
Everything works fine, except that the imageViewB in the combined bitmap is not positioned appropriately (see the attached picture) 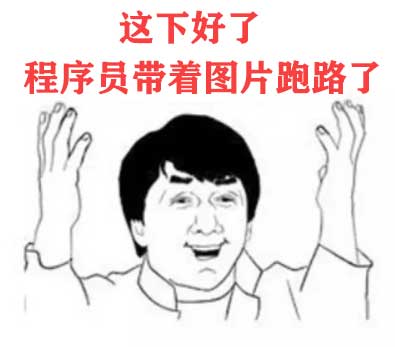
一切正常,除了组合位图中的imageViewB没有正确定位(参见附图)
Code (MainActivity.java):
public class MainActivity extends ActionBarActivity {
Bitmap bmMask = null;
Bitmap bmOriginal = null;
Bitmap bmCombined = null;
ImageView normalImgView;
ImageView maskImgView;
ImageView combinedImgView;
static final int REQUEST_TAKE_PHOTO = 55;
static final int REQUEST_LOAD_IMAGE = 60;
String currentImagePath;
static final String TAG = "mover";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
combinedImgView = (ImageView) findViewById(R.id.combinedImgView);
normalImgView = (ImageView) findViewById(R.id.normalImgView);
maskImgView = (ImageView) findViewById(R.id.maskImgView);
bmMask = ((BitmapDrawable) maskImgView.getDrawable()).getBitmap();
Button addBtn = (Button) findViewById(R.id.addBtn);
addBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
bmCombined = ProcessingBitmap();
if(bmCombined!=null){
combinedImgView.setImageBitmap(bmCombined);
}
else {
Log.d(MainActivity.TAG, "combined bm is null");
}
}
});
Button button = (Button) findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Log.d(MainActivity.TAG, "clicked");
getImageFromCameraIntent();
}
});
MultiTouchListener mTouchListener = new MultiTouchListener();
mTouchListener.isRotateEnabled = false;
// mTouchListener.isTranslateEnabled = false;
mTouchListener.isScaleEnabled = false;
maskImgView.setOnTouchListener(mTouchListener);
}
// take image from camera
private void getImageFromGalleryIntent() {
Intent i = new Intent(Intent.ACTION_PICK, android.provider.MediaStore.Images.Media.EXTERNAL_CONTENT_URI);
startActivityForResult(i, REQUEST_LOAD_IMAGE);
}
// take image from camera
private void getImageFromCameraIntent() {
Intent takePictureIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
// Ensure that there's a camera activity to handle the intent
if (takePictureIntent.resolveActivity(this.getPackageManager()) != null) {
// Create the File where the photo should go
File photoFile = null;
try {
photoFile = createImageFile();
} catch (IOException ex) {
// Error occurred while creating the File
}
// Continue only if the File was successfully created
if (photoFile != null) {
takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT,
Uri.fromFile(photoFile));
startActivityForResult(takePictureIntent, REQUEST_TAKE_PHOTO);
}
}
}
// create a image file for storing full size image taken from camera
private File createImageFile() throws IOException {
// Create an image file name
String timeStamp = new SimpleDateFormat("yyyyMMdd_HHmmss").format(new Date());
String imageFileName = timeStamp + "_";
File storageDir = Environment.getExternalStoragePublicDirectory(
Environment.DIRECTORY_PICTURES);
File image = File.createTempFile(
imageFileName, /* prefix */
".jpg", /* suffix */
storageDir /* directory */
);
// Save a file: path for use with ACTION_VIEW intents
currentImagePath = image.getAbsolutePath();
Log.d(MainActivity.TAG, "photo path: " + currentImagePath);
return image;
}
@Override
public void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == REQUEST_TAKE_PHOTO && resultCode == Activity.RESULT_OK) {
// photo returned from camera
// update UI
updatePhotoView(currentImagePath);
} else if (requestCode == REQUEST_LOAD_IMAGE && resultCode == Activity.RESULT_OK) {
// photo returned from gallery
// update UI
Uri selectedImage = data.getData();
String[] filePathColumn = {MediaStore.Images.Media.DATA};
Cursor cursor = this.getContentResolver().query(selectedImage, filePathColumn, null, null, null);
cursor.moveToFirst();
int columnIndex = cursor.getColumnIndex(filePathColumn[0]);
String picturePath = cursor.getString(columnIndex);
cursor.close();
updatePhotoView(picturePath);
} else {
Toast.makeText(this, "Cancelled", Toast.LENGTH_LONG).show();
}
}
// scale down the image, then display it to image view
private void updatePhotoView(String photoPath) {
// Get the dimensions of the bitmap
BitmapFactory.Options bmOptiOns= new BitmapFactory.Options();
bmOptions.inJustDecodeBounds = true;
BitmapFactory.decodeFile(photoPath, bmOptions);
// Decode the image file into a Bitmap sized to fill the View
bmOptions.inJustDecodeBounds = false;
bmOptions.inPurgeable = true;
Bitmap bitmap = BitmapFactory.decodeFile(photoPath, bmOptions);
bmOriginal = bitmap;
normalImgView.setImageBitmap(bmOriginal);
}
private Bitmap ProcessingBitmap(){
Bitmap newBitmap = null;
int w;
w = bmOriginal.getWidth();
int h;
h = bmOriginal.getHeight();
Bitmap.Config cOnfig= bmOriginal.getConfig();
if(cOnfig== null){
cOnfig= Bitmap.Config.ARGB_8888;
}
newBitmap = Bitmap.createBitmap(w, h, config);
Canvas newCanvas = new Canvas(newBitmap);
newCanvas.drawBitmap(bmOriginal, 0, 0, null);
Paint paint = new Paint();
float left = maskImgView.getLeft();
float top = maskImgView.getTop();
Log.d(MainActivity.TAG, "left is " + left);
Log.d(MainActivity.TAG, "top is " + top);
newCanvas.drawBitmap(bmMask, left, top, paint);
return newBitmap;
}
}
Code (xml)
My Thought:
I guess the positioning issue occurs in the few lines of code listed below. I just have no idea how to get it right, yet.
我想定位问题出现在下面列出的几行代码中。我只是不知道如何做到这一点。
float left = maskImgView.getLeft();
float top = maskImgView.getTop();
newCanvas.drawBitmap(bmMask, left, top, paint);
Any help is appreciated!
任何帮助表示赞赏!
1 个解决方案